mirror of
https://github.com/jkriege2/JKQtPlotter.git
synced 2024-12-25 10:01:38 +08:00
added example for MathImagePLot
This commit is contained in:
parent
9988df1506
commit
9ff25f4b0d
114
README.md
114
README.md
@ -83,6 +83,7 @@ int main(int argc, char* argv[])
|
|||||||
}
|
}
|
||||||
```
|
```
|
||||||
The result looks like this:
|
The result looks like this:
|
||||||
|
|
||||||

|

|
||||||
|
|
||||||
### Simple line-graph with error bars
|
### Simple line-graph with error bars
|
||||||
@ -369,29 +370,110 @@ If you use `JKQTPbarHorizontalGraphStacked` instead of `JKQTPbarVerticalStackabl
|
|||||||
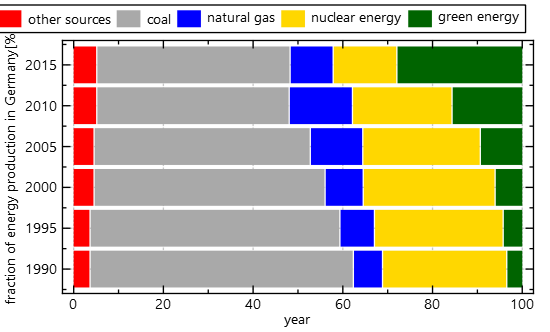
|
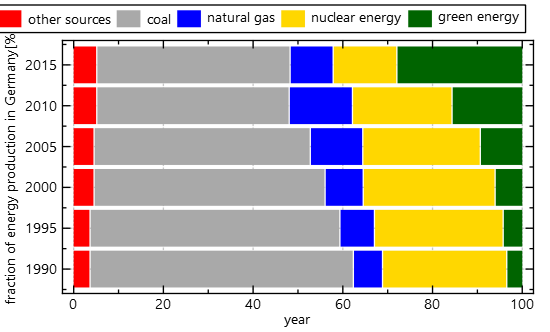
|
||||||
|
|
||||||
|
|
||||||
## Screenshots
|
|
||||||
### Scatter Plots and Boxplots
|
|
||||||

|
|
||||||
|
|
||||||
### Different Types of Barcharts
|
### Simple math image plot
|
||||||

|
This project (see `./test/jkqtplotter_simpletest_imageplot/`) simply creates a JKQtPlotter widget (as a new window) and adds a color-coded image plot of a mathematical function (here the Airy disk). The image is stored as a simple C-array in row-major ordering and then copied into a single column of the internal datasdtore (JKQTPMathImage could be directly used without the internal datastore). This very simple interface can also be used to interface with many common image processing libraries, like CImg or OpenCV.
|
||||||
|
|
||||||
### Image Plots
|
The soruce code of the main application is (see `./test/jkqtplotter_simpletest_imageplot/jkqtplotter_simpletest_imageplot.cpp`):
|
||||||
You can plot C-arrays as images in different color-coding styles. Diferent Overlays/masks are also available. Finally you can use LaTeX markup to format any axis/plot/tick/... label. there is an internal LaTeX parser in this package.
|
```c++
|
||||||

|
#include <QApplication>
|
||||||
|
#include <cmath>
|
||||||
|
#include "jkqtplotter/jkqtplotter.h"
|
||||||
|
#include "jkqtplotter/jkqtpimageelements.h"
|
||||||
|
|
||||||
|
#ifndef M_PI
|
||||||
|
#define M_PI 3.14159265358979323846
|
||||||
|
#endif
|
||||||
|
|
||||||
|
|
||||||
### Plotting a user-defined (parsed) function
|
int main(int argc, char* argv[])
|
||||||
Yes, a complete math expression parser is contained!
|
{
|
||||||

|
QApplication app(argc, argv);
|
||||||
|
|
||||||
### Axis-Label styles in LogLog-Plot
|
JKQtPlotter plot;
|
||||||

|
// 1. create a plotter window and get a pointer to the internal datastore (for convenience)
|
||||||
|
plot.get_plotter()->set_useAntiAliasingForGraphs(true); // nicer (but slower) plotting
|
||||||
|
plot.get_plotter()->set_useAntiAliasingForSystem(true); // nicer (but slower) plotting
|
||||||
|
plot.get_plotter()->set_useAntiAliasingForText(true); // nicer (but slower) text rendering
|
||||||
|
JKQTPdatastore* ds=plot.getDatastore();
|
||||||
|
|
||||||
|
// 2. now we create data for the charts (taken from https://commons.wikimedia.org/wiki/File:Energiemix_Deutschland.svg)
|
||||||
|
const int NX=100; // image dimension in x-direction [pixels]
|
||||||
|
const int NY=100; // image dimension in x-direction [pixels]
|
||||||
|
const double dx=1e-2; // size of a pixel in x-direction [micrometers]
|
||||||
|
const double dy=1e-2; // size of a pixel in x-direction [micrometers]
|
||||||
|
const double w=static_cast<double>(NX)*dx;
|
||||||
|
const double h=static_cast<double>(NY)*dy;
|
||||||
|
double airydisk[NX*NY]; // row-major image
|
||||||
|
|
||||||
|
// 2.1 Parameters for airy disk plot (see https://en.wikipedia.org/wiki/Airy_disk)
|
||||||
|
double NA=1.1; // numerical aperture of lens
|
||||||
|
double wavelength=488e-3; // wavelength of the light [micrometers]
|
||||||
|
|
||||||
|
// 2.2 calculate image of airy disk in a row-major array
|
||||||
|
double x, y=-h/2.0;
|
||||||
|
for (int iy=0; iy<NY; iy++ ) {
|
||||||
|
x=-w/2.0;
|
||||||
|
for (int ix=0; ix<NX; ix++ ) {
|
||||||
|
const double r=sqrt(x*x+y*y);
|
||||||
|
const double v=2.0*M_PI*NA*r/wavelength;
|
||||||
|
airydisk[iy*NX+ix] = pow(2.0*j1(v)/v, 2);
|
||||||
|
x+=dx;
|
||||||
|
}
|
||||||
|
y+=dy;
|
||||||
|
}
|
||||||
|
|
||||||
|
|
||||||
### Parametrized Scatter Plots and Data Viewer
|
|
||||||
Scatter Plots can have symbols where the shape/color/size is parametrized by a data column. Also the plotter is built around an internal datastore, which you can access (readonly!!!) by a data-viewer that is accessible from the contextmenu in any plot.
|
// 3. make data available to JKQtPlotter by adding it to the internal datastore.
|
||||||
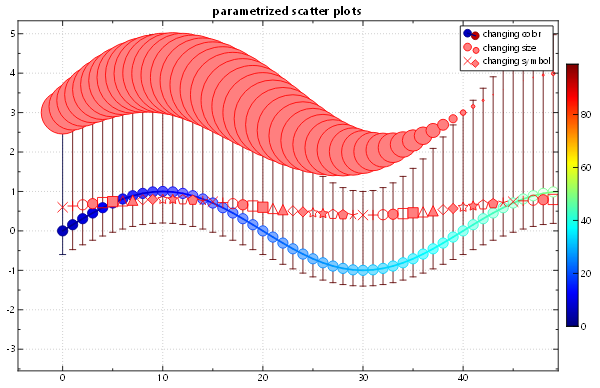
|
// Note: In this step the data is copied (of not specified otherwise)
|
||||||
|
// the variables cYear, cOther ... will contain the internal column ID of the
|
||||||
|
// newly created columns with names "year" and "other" ... and the (copied) data
|
||||||
|
size_t cAiryDisk=ds->addCopiedImageAsColumn(airydisk, NX, NY, "imagedata");
|
||||||
|
|
||||||
|
// 4. create graphs in the plot, which plots the dataset year/other, year/coal, ...
|
||||||
|
// The color of the graphs is set by calling set_fillColor_and_darkenedColor(), which sets the
|
||||||
|
// fillColor to the given color and makes the outline of the bars (i.e. their "color") a darker
|
||||||
|
// shade of the given color.
|
||||||
|
JKQTPColumnMathImage* graph=new JKQTPColumnMathImage(&plot);
|
||||||
|
graph->set_title("");
|
||||||
|
graph->set_imageColumn(cAiryDisk);
|
||||||
|
graph->set_Nx(NX);
|
||||||
|
graph->set_Ny(NY);
|
||||||
|
graph->set_x(-w/2.0);
|
||||||
|
graph->set_y(-h/2.0);
|
||||||
|
graph->set_width(w);
|
||||||
|
graph->set_height(h);
|
||||||
|
graph->set_palette(JKQTPMathImageMATLAB); // color-map is "MATLAB"
|
||||||
|
graph->get_colorBarRightAxis()->set_axisLabel("light intensity [A.U.]"); // get coordinate axis of color-bar and set its label
|
||||||
|
|
||||||
|
// 5. add the graphs to the plot, so it is actually displayed
|
||||||
|
plot.addGraph(graph);
|
||||||
|
|
||||||
|
// 6. set axis labels
|
||||||
|
plot.get_xAxis()->set_axisLabel("x [{\\mu}m]");
|
||||||
|
plot.get_yAxis()->set_axisLabel("y [{\\mu}m]");
|
||||||
|
|
||||||
|
// 7. fix axis and plot aspect ratio to 1
|
||||||
|
plot.get_plotter()->set_maintainAspectRatio(true);
|
||||||
|
plot.get_plotter()->set_maintainAxisAspectRatio(true);
|
||||||
|
|
||||||
|
// 8 autoscale the plot so the graph is contained
|
||||||
|
plot.zoomToFit();
|
||||||
|
|
||||||
|
// show plotter and make it a decent size
|
||||||
|
plot.show();
|
||||||
|
plot.resize(600,600);
|
||||||
|
plot.setWindowTitle("JKQTPColumnMathImage");
|
||||||
|
|
||||||
|
|
||||||
|
return app.exec();
|
||||||
|
}
|
||||||
|
```
|
||||||
|
The result looks like this:
|
||||||
|
|
||||||
|

|
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
@ -9,8 +9,11 @@
|
|||||||
|
|
||||||
## Image Plots
|
## Image Plots
|
||||||
You can plot C-arrays as images in different color-coding styles. Diferent Overlays/masks are also available. Finally you can use LaTeX markup to format any axis/plot/tick/... label. there is an internal LaTeX parser in this package.
|
You can plot C-arrays as images in different color-coding styles. Diferent Overlays/masks are also available. Finally you can use LaTeX markup to format any axis/plot/tick/... label. there is an internal LaTeX parser in this package.
|
||||||
|
|
||||||

|

|
||||||
|
|
||||||
|

|
||||||
|
|
||||||
|
|
||||||
## Plotting a user-defined (parsed) function
|
## Plotting a user-defined (parsed) function
|
||||||
Yes, a complete math expression parser is contained!
|
Yes, a complete math expression parser is contained!
|
||||||
@ -22,9 +25,11 @@ Yes, a complete math expression parser is contained!
|
|||||||
|
|
||||||
## Parametrized Scatter Plots and Data Viewer
|
## Parametrized Scatter Plots and Data Viewer
|
||||||
Scatter Plots can have symbols where the shape/color/size is parametrized by a data column. Also the plotter is built around an internal datastore, which you can access (readonly!!!) by a data-viewer that is accessible from the contextmenu in any plot.
|
Scatter Plots can have symbols where the shape/color/size is parametrized by a data column. Also the plotter is built around an internal datastore, which you can access (readonly!!!) by a data-viewer that is accessible from the contextmenu in any plot.
|
||||||
|
|
||||||
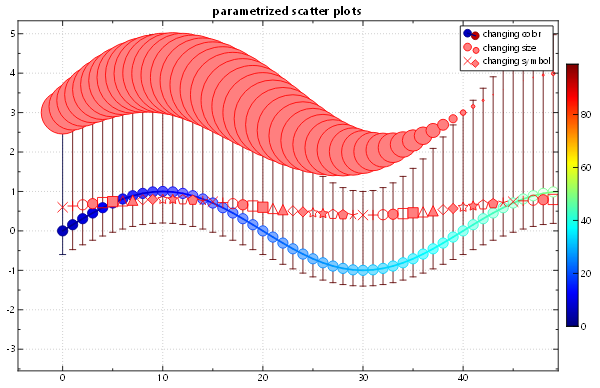
|
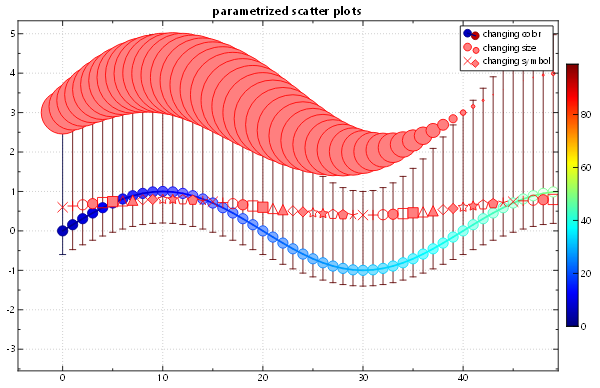
|
||||||
|
|
||||||
## Barcharts
|
## Barcharts
|
||||||
|
|
||||||
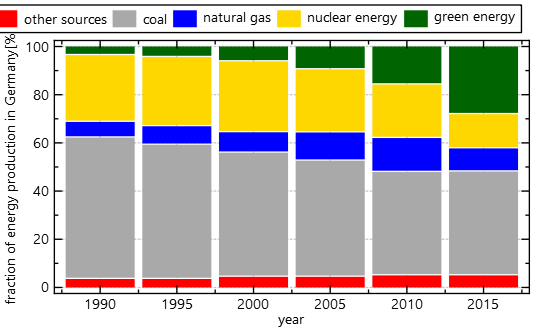
|
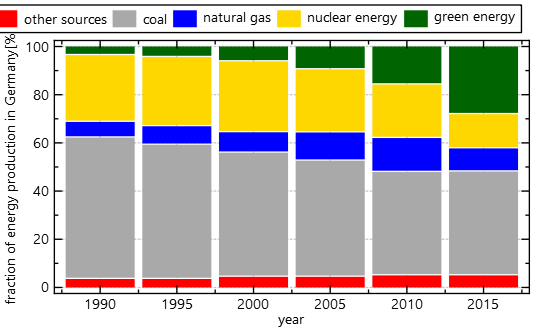
|
||||||
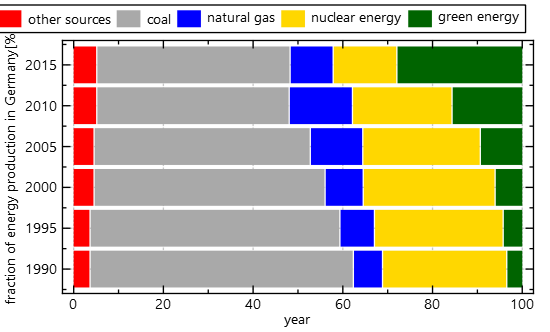
|
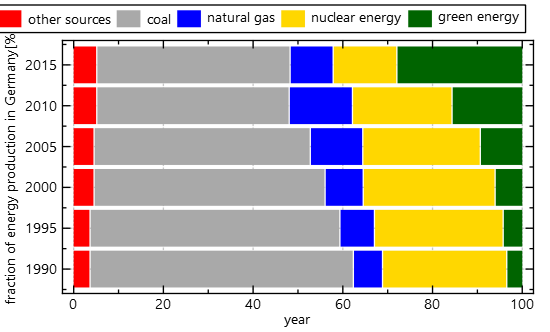
|
||||||

|

|
||||||
|
BIN
screenshots/jkqtplotter_simpletest_imageplot.png
Normal file
BIN
screenshots/jkqtplotter_simpletest_imageplot.png
Normal file
Binary file not shown.
After Width: | Height: | Size: 15 KiB |
@ -0,0 +1,93 @@
|
|||||||
|
#include <QApplication>
|
||||||
|
#include <cmath>
|
||||||
|
#include "jkqtplotter/jkqtplotter.h"
|
||||||
|
#include "jkqtplotter/jkqtpimageelements.h"
|
||||||
|
|
||||||
|
#ifndef M_PI
|
||||||
|
#define M_PI 3.14159265358979323846
|
||||||
|
#endif
|
||||||
|
|
||||||
|
|
||||||
|
int main(int argc, char* argv[])
|
||||||
|
{
|
||||||
|
QApplication app(argc, argv);
|
||||||
|
|
||||||
|
JKQtPlotter plot;
|
||||||
|
// 1. create a plotter window and get a pointer to the internal datastore (for convenience)
|
||||||
|
plot.get_plotter()->set_useAntiAliasingForGraphs(true); // nicer (but slower) plotting
|
||||||
|
plot.get_plotter()->set_useAntiAliasingForSystem(true); // nicer (but slower) plotting
|
||||||
|
plot.get_plotter()->set_useAntiAliasingForText(true); // nicer (but slower) text rendering
|
||||||
|
JKQTPdatastore* ds=plot.getDatastore();
|
||||||
|
|
||||||
|
// 2. now we create data for the charts (taken from https://commons.wikimedia.org/wiki/File:Energiemix_Deutschland.svg)
|
||||||
|
const int NX=100; // image dimension in x-direction [pixels]
|
||||||
|
const int NY=100; // image dimension in x-direction [pixels]
|
||||||
|
const double dx=1e-2; // size of a pixel in x-direction [micrometers]
|
||||||
|
const double dy=1e-2; // size of a pixel in x-direction [micrometers]
|
||||||
|
const double w=static_cast<double>(NX)*dx;
|
||||||
|
const double h=static_cast<double>(NY)*dy;
|
||||||
|
double airydisk[NX*NY]; // row-major image
|
||||||
|
|
||||||
|
// 2.1 Parameters for airy disk plot (see https://en.wikipedia.org/wiki/Airy_disk)
|
||||||
|
double NA=1.1; // numerical aperture of lens
|
||||||
|
double wavelength=488e-3; // wavelength of the light [micrometers]
|
||||||
|
|
||||||
|
// 2.2 calculate image of airy disk in a row-major array
|
||||||
|
double x, y=-h/2.0;
|
||||||
|
for (int iy=0; iy<NY; iy++ ) {
|
||||||
|
x=-w/2.0;
|
||||||
|
for (int ix=0; ix<NX; ix++ ) {
|
||||||
|
const double r=sqrt(x*x+y*y);
|
||||||
|
const double v=2.0*M_PI*NA*r/wavelength;
|
||||||
|
airydisk[iy*NX+ix] = pow(2.0*j1(v)/v, 2);
|
||||||
|
x+=dx;
|
||||||
|
}
|
||||||
|
y+=dy;
|
||||||
|
}
|
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
// 3. make data available to JKQtPlotter by adding it to the internal datastore.
|
||||||
|
// Note: In this step the data is copied (of not specified otherwise)
|
||||||
|
// the variables cYear, cOther ... will contain the internal column ID of the
|
||||||
|
// newly created columns with names "year" and "other" ... and the (copied) data
|
||||||
|
size_t cAiryDisk=ds->addCopiedImageAsColumn(airydisk, NX, NY, "imagedata");
|
||||||
|
|
||||||
|
// 4. create graphs in the plot, which plots the dataset year/other, year/coal, ...
|
||||||
|
// The color of the graphs is set by calling set_fillColor_and_darkenedColor(), which sets the
|
||||||
|
// fillColor to the given color and makes the outline of the bars (i.e. their "color") a darker
|
||||||
|
// shade of the given color.
|
||||||
|
JKQTPColumnMathImage* graph=new JKQTPColumnMathImage(&plot);
|
||||||
|
graph->set_title("");
|
||||||
|
graph->set_imageColumn(cAiryDisk);
|
||||||
|
graph->set_Nx(NX);
|
||||||
|
graph->set_Ny(NY);
|
||||||
|
graph->set_x(-w/2.0);
|
||||||
|
graph->set_y(-h/2.0);
|
||||||
|
graph->set_width(w);
|
||||||
|
graph->set_height(h);
|
||||||
|
graph->set_palette(JKQTPMathImageMATLAB); // color-map is "MATLAB"
|
||||||
|
graph->get_colorBarRightAxis()->set_axisLabel("light intensity [A.U.]"); // get coordinate axis of color-bar and set its label
|
||||||
|
|
||||||
|
// 5. add the graphs to the plot, so it is actually displayed
|
||||||
|
plot.addGraph(graph);
|
||||||
|
|
||||||
|
// 6. set axis labels
|
||||||
|
plot.get_xAxis()->set_axisLabel("x [{\\mu}m]");
|
||||||
|
plot.get_yAxis()->set_axisLabel("y [{\\mu}m]");
|
||||||
|
|
||||||
|
// 7. fix axis and plot aspect ratio to 1
|
||||||
|
plot.get_plotter()->set_maintainAspectRatio(true);
|
||||||
|
plot.get_plotter()->set_maintainAxisAspectRatio(true);
|
||||||
|
|
||||||
|
// 8 autoscale the plot so the graph is contained
|
||||||
|
plot.zoomToFit();
|
||||||
|
|
||||||
|
// show plotter and make it a decent size
|
||||||
|
plot.show();
|
||||||
|
plot.resize(600,600);
|
||||||
|
plot.setWindowTitle("JKQTPColumnMathImage");
|
||||||
|
|
||||||
|
|
||||||
|
return app.exec();
|
||||||
|
}
|
@ -0,0 +1,16 @@
|
|||||||
|
# source code for this simple demo
|
||||||
|
SOURCES = jkqtplotter_simpletest_imageplot.cpp
|
||||||
|
|
||||||
|
# configure Qt
|
||||||
|
CONFIG += qt
|
||||||
|
QT += core gui svg
|
||||||
|
greaterThan(QT_MAJOR_VERSION, 4): QT += widgets printsupport
|
||||||
|
|
||||||
|
# output executable name
|
||||||
|
TARGET = jkqtplotter_simpletest_imageplot
|
||||||
|
|
||||||
|
# include JKQtPlotter source code
|
||||||
|
include(../../lib/jkqtplotter.pri)
|
||||||
|
# here you can activate some debug options
|
||||||
|
#DEFINES += SHOW_JKQTPLOTTER_DEBUG
|
||||||
|
#DEFINES += JKQTBP_AUTOTIMER
|
Loading…
Reference in New Issue
Block a user